Welcome to Rhinestone
Get familiar with Rhinestone’s tech and figure out where to start
Rhinestone is the simplest way to build powerful self-custodial Web3 applications. We remove the complexities of smart accounts and chains (via chain abstraction) so that you can focus on what matters to your users.
Rhinestone is a smart account platform powered by intents. We provide infrastructure and tooling to build on any compatible smart account, extend the account’s features with modules, or perform near-instant crosschain intents.
Rhinestone is the ideal tech stack for developers to build web3 apps with seamless UX.
Get Started
Choose your path to learning how to build on chain-abstracted smart accounts with Rhinestone.
Build on Smart Accounts
Embed smart accounts into your wallet/application or upgrade an EOA (via EIP-7702) through our simple SDK. Enable simple user onboarding, abstract away gas for your users, and enable permissions via Smart Sessions to deliver one-click experiences or self-custodial automations.
Start with our Quickstart guide and progress through the SDK to learn how to enable an omnichain smart account application.
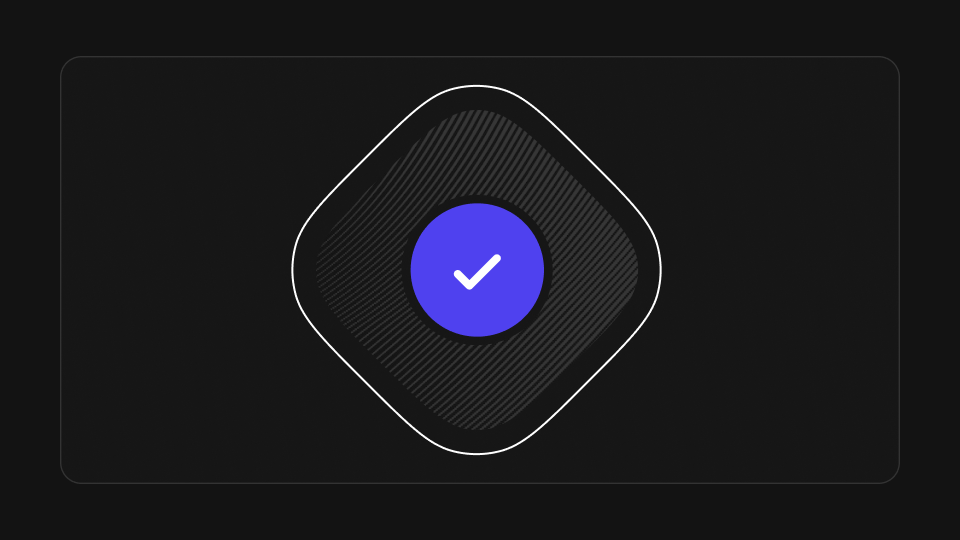
Quickstart
Set up a smart account and submit your first crosschain intent
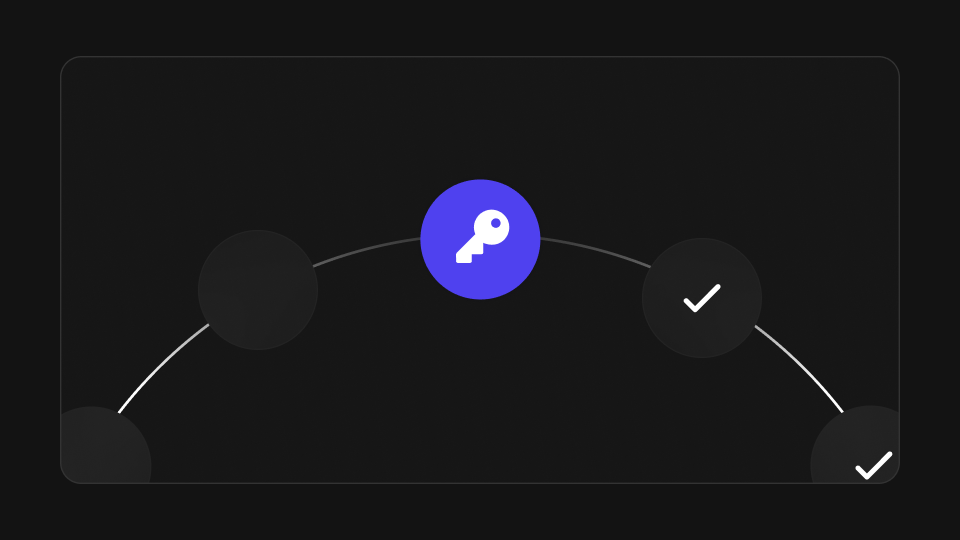
Smart Sessions
Create onchain permissions for self-custodial automation and 1-click UX
Integrate Chain Abstraction
Rhinestone’s transaction infrastructure is powered by intents. Whether your users are transacting on the same chain or crosschain, you only need to integrate one API and make one simple request - we call these transactions “omnichain transactions”.
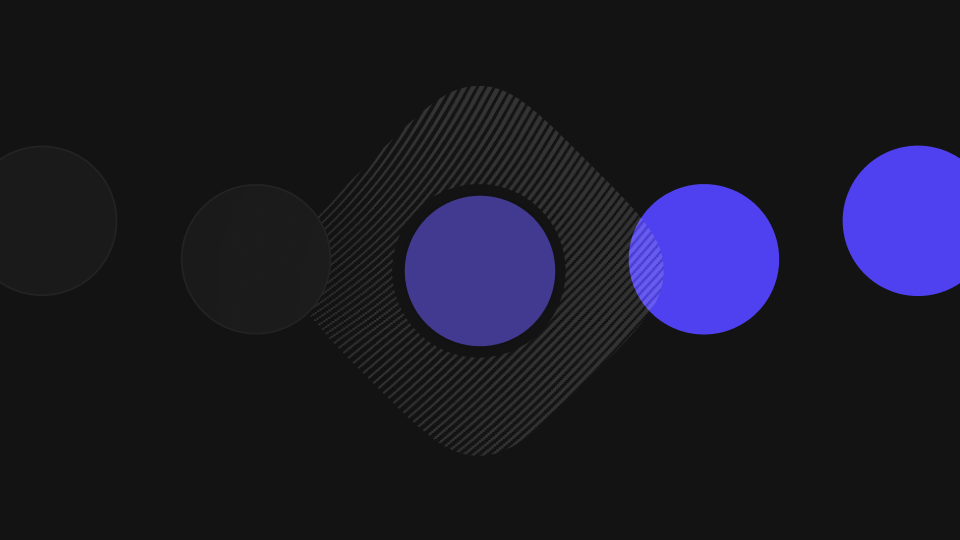
Omnichain Transactions
Embed crosschain intents into your application
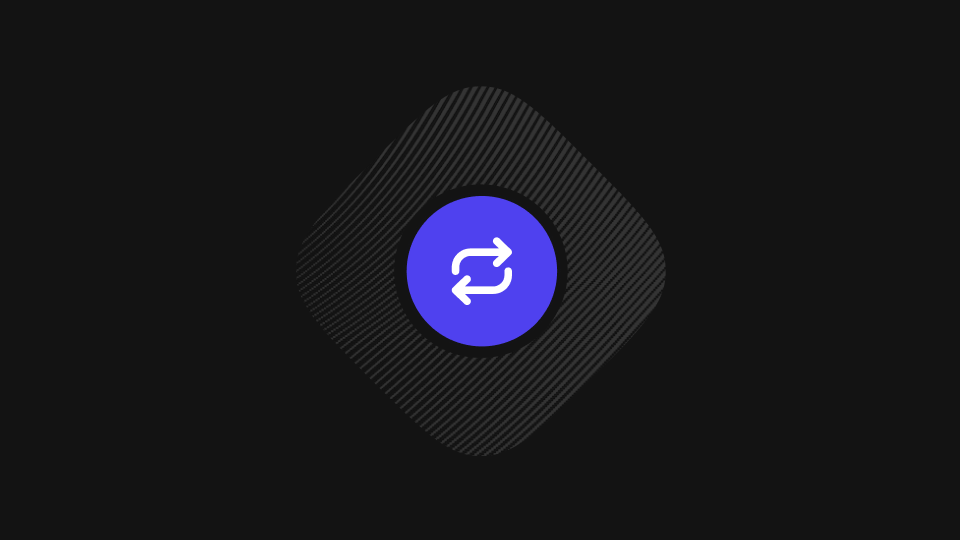
Omnichain Swaps
Perform instant crosschain swap intents
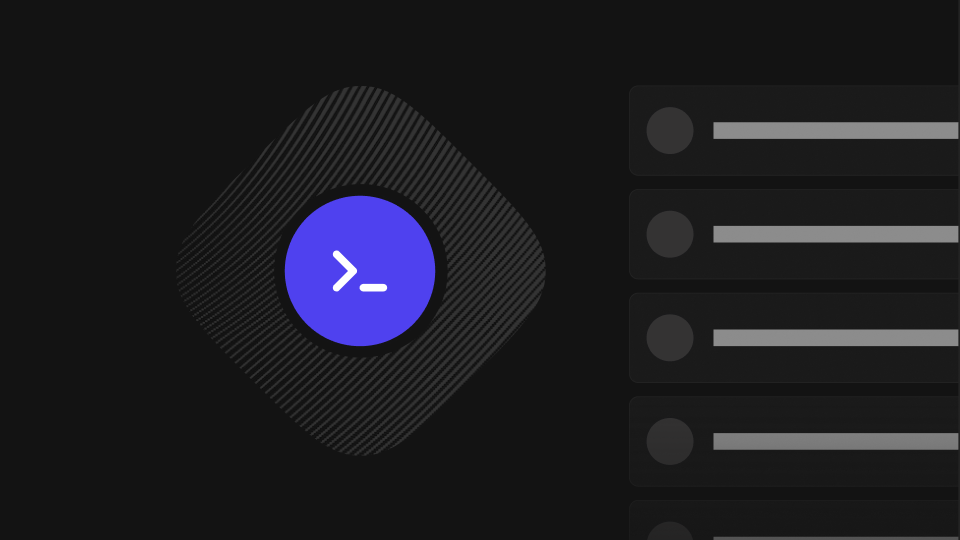
API Reference
Endpoints, methods, parameters, and responses of the Orchestrator API
Build Modules
Rhinestone wrote the standard for modular smart accounts: ERC-7579, a minimal standard for modular smart accounts. This is the foundation for Rhinestone’s account model. The major benefit of modularity is composability and extensibility.
If you’re looking to customize your account beyond our core features, check out ModuleKit and our extensive library of modules.
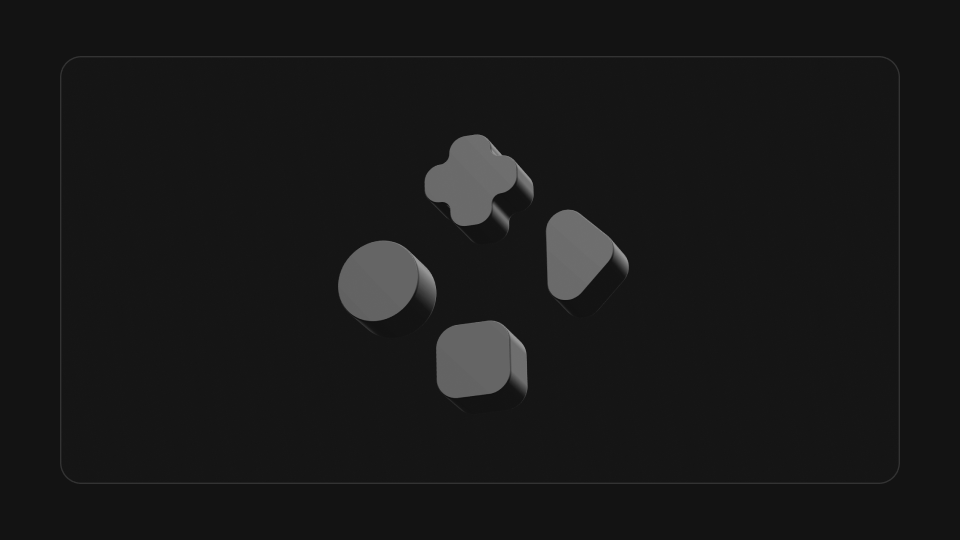
ModuleKit
Build custom components to extend the feature set of your smart wallet
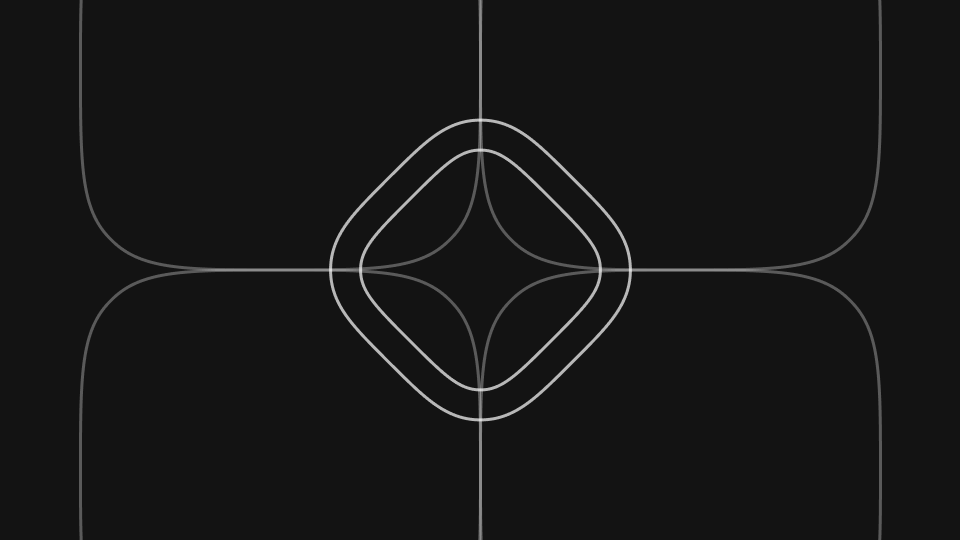
Module Template
Use our templates to get started with building modules
Learn How Rhinestone Works
Explore Rhinestone in depth and discover the components that make Rhinestone’s unique smart account offering possible.
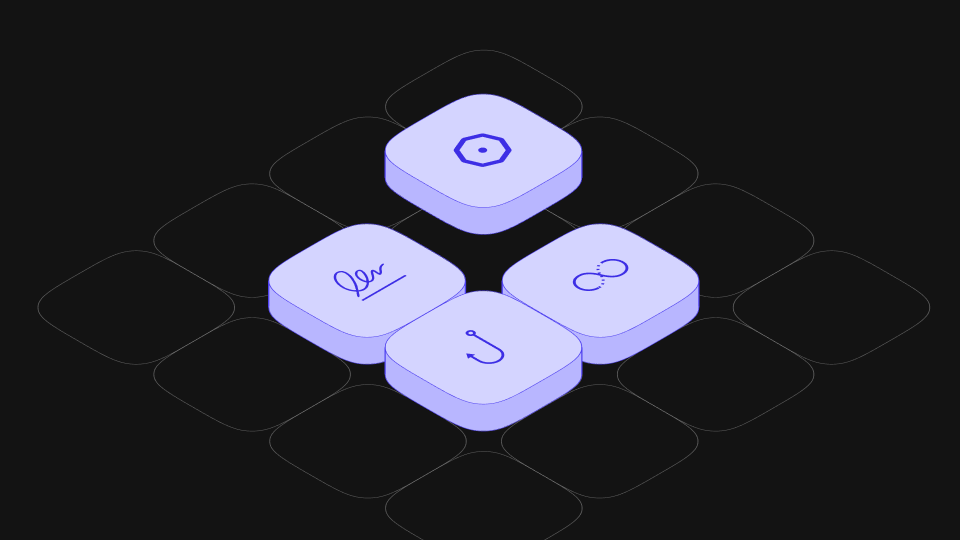
Modular Smart Accounts
Understand Rhinestone’s underlying account model
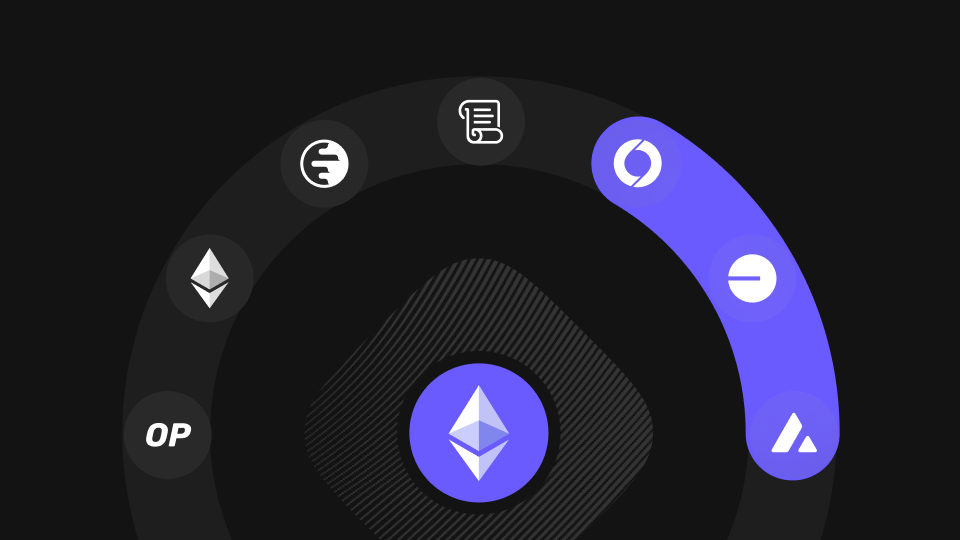
Chain Abstraction
Deep dive into Rhinestone’s chain abstraction offering
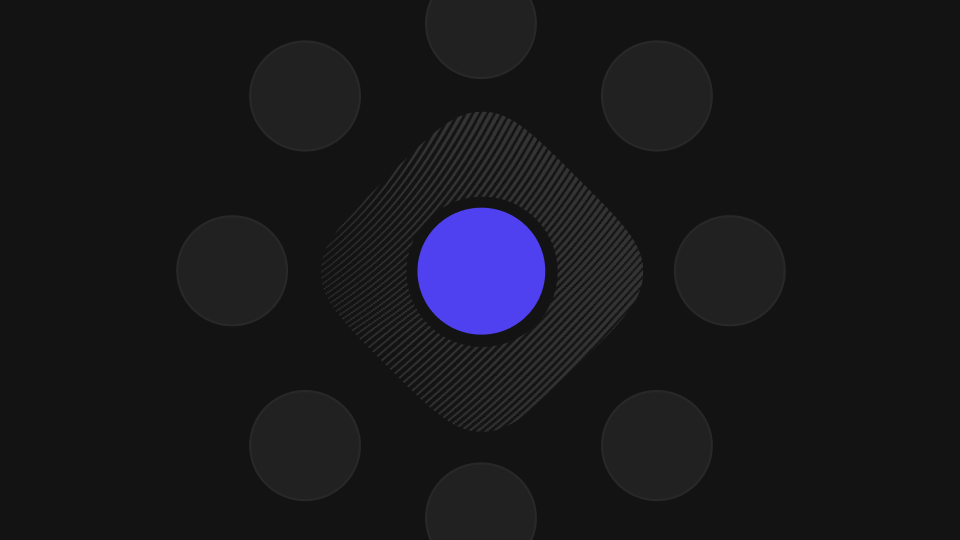
What are Intents?
Learn about intents and the Rhinestone intent flow
Developer Support
For more support, reach out on Telegram so that we can spin up a private channel.